Unit testing is an essential practice in software development that allows developers to verify the correctness of individual components or units of their code. In Node.js, unit testing is crucial in ensuring the reliability and maintainability of applications.
This article will help you understand the basics, best practices, and useful tools for writing effective and robust unit tests in Node.js.
Moreover, you can learn how ThinkPalm’s dedicated software developers use unit testing processes to ensure flawless software development and reliable and maintainable applications.
Unit Testing in Node.js: An Overview
Unit testing is a software testing technique that focuses on verifying the correctness of individual components or units of a software system. Units refer to the smallest testable parts of an application, such as functions, methods, or classes. Unit tests are designed to isolate these units from the rest of the system and test them in isolation to ensure they behave as expected and meet the specified requirements.
Why is Unit Testing Important?
Unit testing is a crucial aspect of software development, and its importance stems from several reasons:
- Early Bug Detection: Unit testing helps detect bugs and issues early in the development cycle. By testing individual units in isolation, developers can identify and fix problems before propagating to other system parts, making debugging and troubleshooting more manageable.
- Improved Code Quality: Writing unit tests forces developers to think through the design and implementation of their code more thoroughly. It promotes writing modular, reusable, and maintainable code by enforcing single responsibility and separation of concerns.
- Regression Testing: Unit tests act as a safety net, providing a means to identify regression issues as software evolves quickly. By running unit tests after each code change, developers can ensure that previously working functionality remains intact.
- Facilitates Refactoring: Unit tests play a crucial role in refactoring by allowing developers to modify and restructure code with confidence. Tests act as a safety net, ensuring that refactored code continues to pass the same set of tests, thereby maintaining the desired behavior.
- Collaboration and Documentation: Unit tests serve as executable documentation for the codebase and promote collaboration within development teams. They provide examples and use cases for using units and their expected behavior.
- Faster Debugging: When a unit test fails, it clearly indicates which unit is causing the problem. This focused feedback helps narrow down the source of the issue, making debugging faster and more efficient.
- Continuous Integration and Deployment: Unit tests are crucial to a robust continuous integration and continuous deployment (CI/CD) pipeline. By automating the execution of unit tests as part of the build process, teams can ensure that new changes do not introduce unintended side effects and that the software remains stable and deployable.
Test-driven development (TDD) vs test-after development
Test-driven development (TDD) and test-after development are two approaches to writing tests in software development. Here’s a quick comparison:
1. Test-After Development:
- Code is written first, and tests are added later.
- Pros: Allows focused code implementation, suitable for evolving requirements or quick prototyping.
- Cons: This may lead to difficult-to-test code, missing edge cases, and time-consuming debugging without proper test coverage.
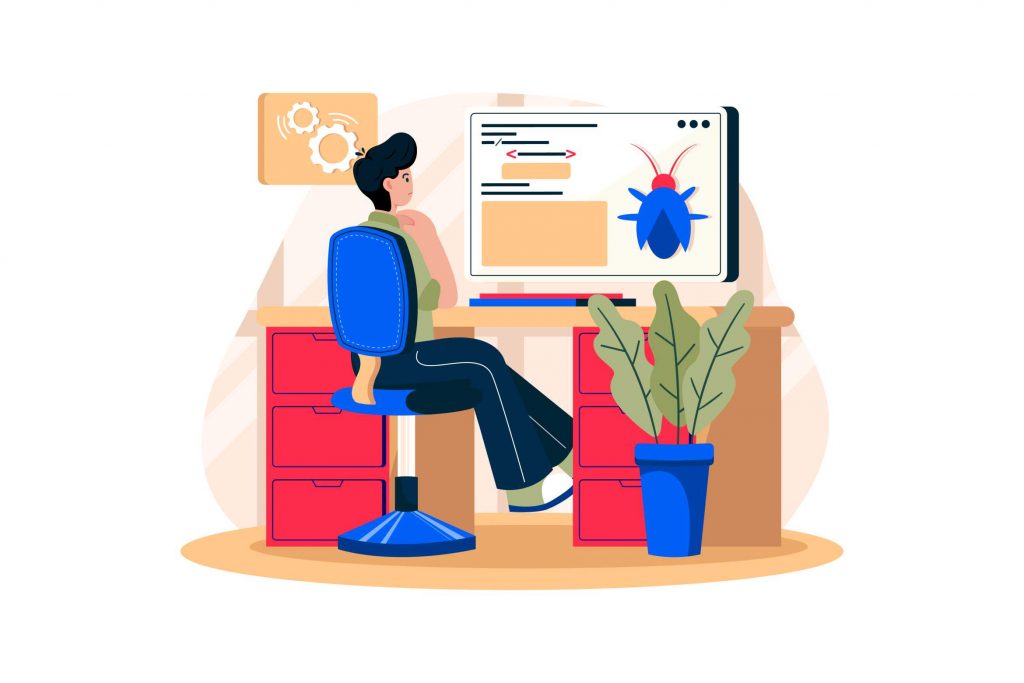
2. Test-Driven Development (TDD):
- Tests are written before implementing code.
- Pros: Ensures better code quality, immediate feedback, and incremental development.
- Cons: Requires upfront time and effort, challenging in unclear or rapidly changing requirements.
How to Choose between TDD and Test-After Development?
- TDD is effective for well-defined requirements and comprehensive test coverage.
- Test after development suits situations with immediate functionality needs or evolving requirements.
- A hybrid approach can be adopted, combining TDD for critical parts and retroactive testing for others.
Ultimately, the goal is to balance test coverage, code quality, and development efficiency. Both TDD and test-after development have their merits, and the choice depends on the specific project and development context.
How to Set Up a Unit Testing Framework?
In Node.js, there are several popular unit testing frameworks to choose from. Some of the widely used frameworks are Mocha, Jest, and NodeUnit. Each framework has its own strengths and features that make it suitable for different project requirements.
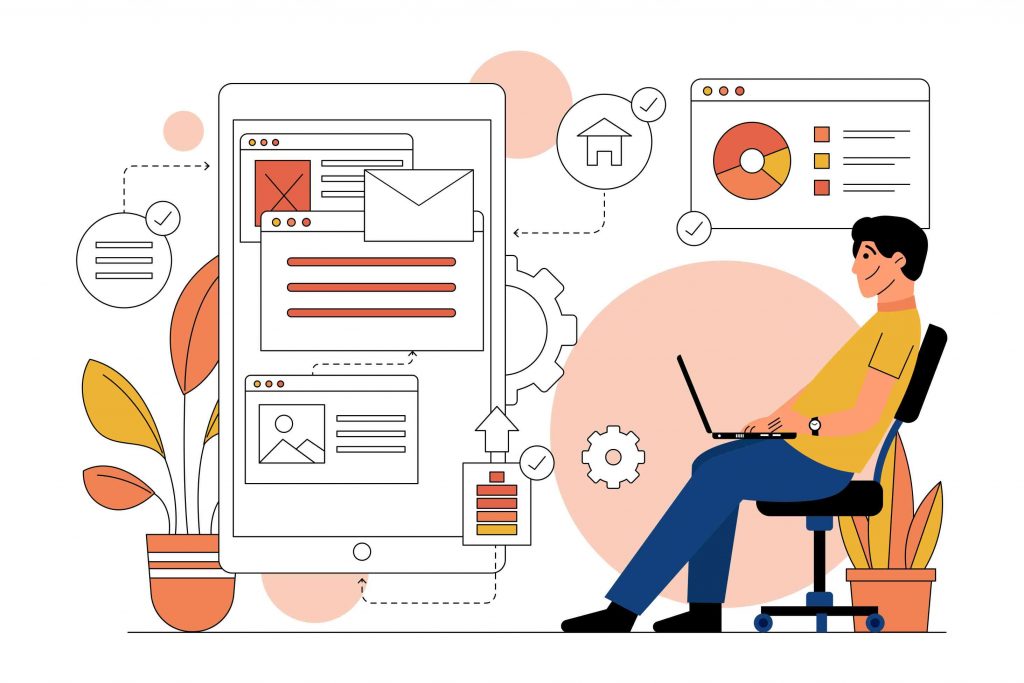
- Mocha: Known for its flexibility, Mocha allows developers to customize their testing workflow. It provides a solid foundation for writing unit tests and can be combined with assertion libraries like Chai for enhanced functionality.
- Jest: Originally developed by Facebook for testing React applications, Jest has gained popularity for its simplicity and “zero configuration” approach. It offers built-in support for mocking, code coverage, and snapshot testing, making it a comprehensive solution for many testing needs.
- NodeUnit: Inspired by JUnit, NodeUnit is a lightweight and simple unit testing framework for Node.js. It provides basic functionality for writing and running tests and is suitable for projects with minimal testing requirements.
A step-by-step guide for installation and configuration
Step 1: Set up a Node.js project
Before installing Mocha and Chai, make sure you have set up a Node.js project. Start by creating a new directory for your project and then navigate to that directory using a terminal or command prompt.
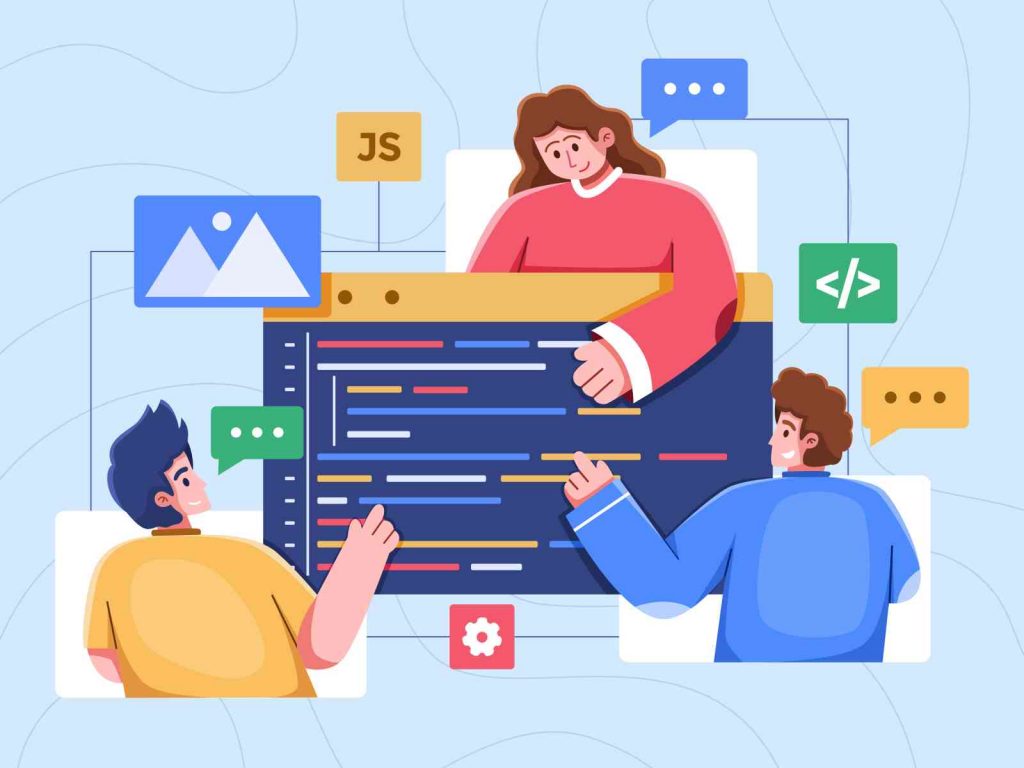
Step 2: Initialize a package.json file
To create a package.json file with default settings in the project directory, you can run the following command:
npm init -y
Executing this command will automatically generate a basic package.json file, saving you the effort of manually configuring the settings.
Step 3: Install Mocha and Chai
Run the following command to install Mocha and Chai as development dependencies:
npm install mocha chai --save-dev
Running this command will install both Mocha and Chai and save them as devDependencies in your package.json file. This ensures that these libraries are specifically meant for development purposes and won’t be included in the production build.
Step 4: Create a test directory
To organize your test files, please navigate to your project directory and create a new folder named “test.” This directory will serve as a dedicated storage space for all your test-related files.
Step 5: Write your tests
Within the test directory, create a new JavaScript file named “test.js” to facilitate your testing process. You can utilize Mocha and Chai frameworks to write your tests. Consider the following example code:
const assert = require('chai').assert;
describe('Array', function() {
describe('#indexOf()', function() {
it('should return -1 when the value is not present', function() {
assert.equal([1, 2, 3].indexOf(4), -1);
});
it('should return the index when the value is present', function() {
assert.equal([1, 2, 3].indexOf(2), 1);
});
});
});
In the provided illustration, Chai’s assert style is employed for conducting assertions. This setup enables you to test various scenarios and validate the desired outcomes efficiently.
Step 6: Configure Mocha (optional)
Mocha provides various configuration options. To create a Mocha configuration file, create a new JavaScript file, e.g.,‘mocha.config.js’, in your project directory. Here’s an example configuration that specifies the test directory and sets the reporter to “spec”:
module.exports = {
spec: 'test/**/*.js',
reporter: 'spec'
};
You can customize this configuration file according to your specific needs and preferences.
Step 7: Run your tests
To execute your tests, run the following command in your project directory:
npx mocha
This command will run all the test files located in the test directory.
That’s it! You have now installed and configured Mocha and Chai for testing JavaScript code. You can proceed to write additional tests in the test directory and execute them using Mocha.
Writing Your First Unit Test
Anatomy of a Unit Test
Identify a specific unit of code you want to test. This can be a function, a class, or a module that performs a distinct function in your application.
Write your test
In your test directory, create a new test file (e.g., myTest.spec.js). Import the necessary dependencies and define a test suite using the testing framework’s syntax. Within the test suite, write individual test cases that cover different scenarios and expected outcomes for the unit being tested.
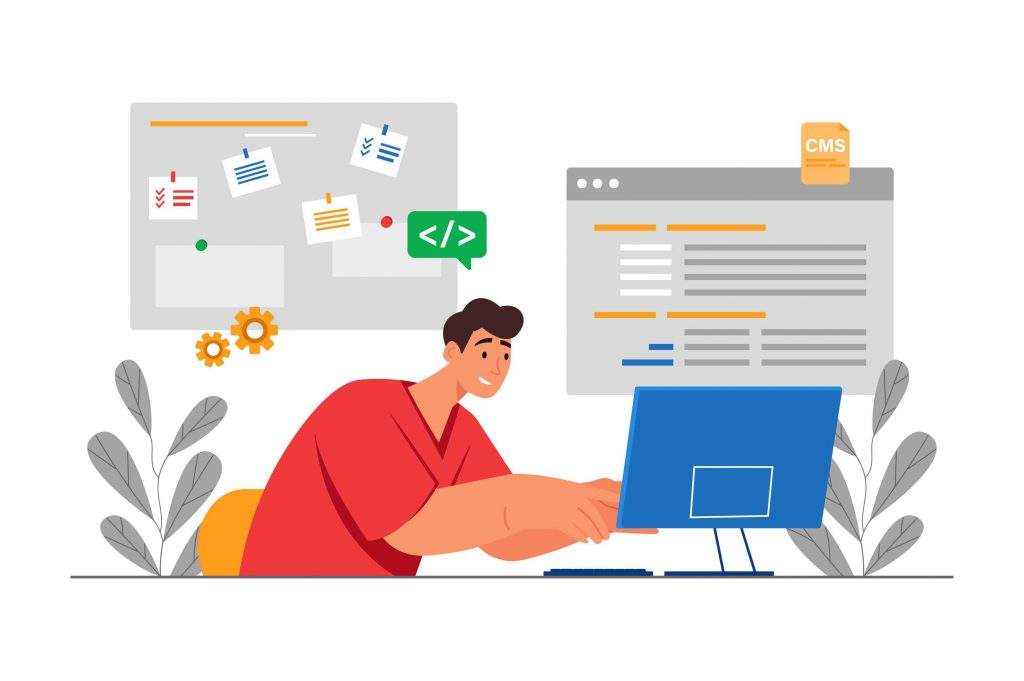
Run the tests
Run your tests using the testing framework’s command-line interface or test runner. For example, with Mocha, you can use the mocha command to execute all test files in the test directory.
Observe the test results
Review the test results to see if all the tests pass successfully. If any tests fail, examine the error messages or stack traces to identify the root cause of the failure.
Repeat and expand
Continue writing more unit tests to cover different parts of your codebase. Aim for comprehensive test coverage to ensure the reliability and maintainability of your software.
Organizing and Structuring Test Suites
- Test Suite Organization: Organizing and structuring test suites is crucial for maintaining a well-organized and maintainable testing codebase. Here are some tips for effectively organizing and structuring your test suites:
- Categorize tests by functionality: Group your tests based on the functionality or feature they are testing. This helps in organizing related tests together, making it easier to locate and understand the purpose of each test suite.
- Use nested describe blocks: Utilize nested describe blocks provided by your testing framework to create a hierarchical structure for your test suites. This helps in organizing tests in a logical and readable manner. For example:
describe('User Management', function() {
describe('User Registration', function() {
// Tests related to user registration
});
describe('User Login', function() {
// Tests related to user login
});
// More describe blocks for other user-related functionality
});
- Separate setup and teardown: If there are common setup and teardown steps required for multiple test suites, extract them into separate before and after hooks or beforeEach and afterEach hooks provided by the testing framework. This ensures consistency and avoids repetition across different test suites.
- Use meaningful and descriptive test names: Give each test a descriptive name that clearly conveys the scenario being tested. This makes it easier to understand the purpose of the test and locate specific tests when needed.
- Create separate files for different modules: If your project has multiple modules or components, consider creating separate test files for each module or component. This helps in keeping the tests focused, maintainable, and easier to manage.
- Use test runners or task runners: Consider using test runners or task runners, such as mocha or jest, that provide features like test file globbing and test parallelization. These tools allow you to define patterns for test files and run tests in parallel, making it easier to manage large test suites.
- Prioritize readability and maintainability: Ensure that your test suites are readable, maintainable, and easily understandable by other developers. Use proper indentation, comments, and meaningful variable names to enhance code clarity.
- Continuously refactor and reorganize: As your project evolves, periodically review and refactor your test suites. This helps in maintaining a clean and well-structured codebase, improving test performance, and ensuring the tests remain relevant to the current state of your application.
Using Assertion Libraries for Assertions
Overview of Assertion Libraries:
When writing tests, using assertion libraries can greatly simplify the process of making assertions and validating expected outcomes. Here are some popular assertion libraries you can use with JavaScript testing frameworks like Mocha and Chai:
1. Chai
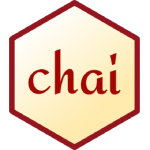
Chai is a flexible assertion library that offers different styles for making assertions, including assert, expect, and should. Chai provides a rich set of assertion methods for various data types and conditions, allowing you to express assertions in a natural and readable manner.
Example with Chai’s expect style:
const { expect } = require('chai');
// Assertion using expect
expect(5).to.be.above(2);
Node.js’s built-in assert: Node.js comes with a built-in assertion library that provides a minimalistic set of assertion methods. It is lightweight and suitable for basic assertions.
Example with Node.js’s assert:
const assert = require('assert');
// Assertion using assert
assert.strictEqual(3 + 2, 5);
2. Jest
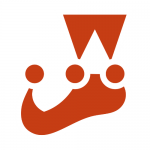
Jest is a popular testing framework that includes its own built-in assertion library. It offers a wide range of assertion methods and provides powerful features for mocking, code coverage, and more.
Example with Jest’s expect:
// Assertion using Jest's expect
expect(10).toBeGreaterThan(5);
3. Should.js
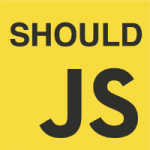
Should.js is another assertion library that provides a fluent and expressive syntax for making assertions. It extends the Object.prototype with a should property, allowing you to chain assertions directly on objects.
Example with Should.js:
require('should');
// Assertion using Should.js
(5).should.be.above(2);
When choosing an assertion library, consider factors like the syntax style you prefer, the level of flexibility and customization needed, and the compatibility with your testing framework of choice. Each library has its own unique features and syntax, so choose the one that best fits your requirements and coding style.
Handling Asynchronous Code in Unit Tests
Dealing with Asynchronous Code:
When writing unit tests for code that includes asynchronous operations, you need to handle the asynchronous nature of the code appropriately. Here are some guidelines for handling asynchronous code in unit tests:
- Use a testing framework that supports asynchronous testing: Make sure your chosen testing framework has built-in support for handling asynchronous code. Most modern testing frameworks, such as Jest for JavaScript provide utilities and syntax for handling asynchronous operations.
- Identify the asynchronous operations in your code: Determine which parts of your code involve asynchronous operations, such as Promises, callbacks, or async/await functions. These are the areas where you’ll need to handle asynchronous code in your unit tests.
- Use callbacks, Promises, or async/await in your tests: Depending on the testing framework and the nature of the asynchronous code you’re testing, you can use callbacks, Promises, or async/await to handle the asynchronous operations. Choose the approach that aligns with your testing framework and coding style.
- Use test-specific techniques to wait for asynchronous operations: When writing unit tests for asynchronous code, you’ll often need to wait for the asynchronous operations to complete before making assertions. Testing frameworks provide various techniques to handle this. For example:
- Callbacks: Pass a callback to the asynchronous function and use the callback to signal test completion.
- Promises: Return the Promise from the asynchronous function and use await or.then() to wait for it to resolve or reject.
- Async/await: Use the await keyword in an async test function to wait for the completion of an asynchronous operation before proceeding with assertions.
- Set appropriate timeouts: Sometimes, asynchronous operations might take longer to complete than expected, and your tests could time out. Set an appropriate timeout duration in your testing framework to avoid false test failures due to timeouts. Consider the expected execution time of the asynchronous operations and choose a timeout that provides sufficient time for completion.
- Handle errors and exceptions: Don’t forget to handle errors and exceptions that might occur during the execution of your asynchronous code. Ensure that your tests catch and handle any errors or exceptions appropriately. Use assertions to check for expected errors or exceptions.
- Consider using mocking and stubbing: In some cases, you might want to isolate the code under test and mock or stub any external dependencies or asynchronous operations. This helps in creating focused tests and allows you to control the behavior of the asynchronous operations for easier testing.
By following these guidelines and utilizing the async/await syntax, you can effectively handle asynchronous code in your unit tests and ensure thorough testing of time-dependent operations.
Mocking and Stubbing Dependencies
Importance of Isolating Dependencies
When writing unit tests, you may encounter situations where your code depends on external dependencies such as databases, APIs, or other modules. To isolate the code under test and create focused tests, you can use mocking and stubbing techniques. Here’s an overview of mocking and stubbing dependencies in unit tests:
Mocking:
Mocking involves creating fake objects or functions that mimic the behavior of the real dependencies but don’t have the actual implementation.
Mocks are used to replace the real dependencies during testing and allow you to control their behavior.
- Mocking frameworks: Many programming languages provide mocking frameworks that make it easier to create mock objects. Examples include Jest (JavaScript)
- Mocking behavior: You can define the expected behavior of a mock object by setting up method calls, return values, exceptions, and other relevant properties. This allows you to simulate different scenarios and ensure your code handles them correctly.
- Verifying interactions: Mocking frameworks usually provide methods to verify that specific methods of the mock object were called with the expected arguments. This helps you ensure that the code under test is interacting correctly with its dependencies.
Stubbing:
Stubbing is similar to mocking, but it focuses on replacing specific methods or functions of a dependency rather than the entire object. Stubs allow you to control the return values or behavior of specific functions to test different code paths.
- Stubbing frameworks: Some mocking frameworks also support stubbing, but you can also create stubs manually by defining functions with desired behavior.
- Stubbing return values: Stubs allow you to define the return values of specific methods or functions. This lets you simulate different scenarios without the need for the real implementation.
- Stubbing behavior: In addition to return values, you can stub behavior such as raising exceptions or triggering side effects to test error handling or specific code paths.
Dependency injection:
Another approach to handling dependencies in unit tests is through dependency injection. Instead of directly instantiating or calling dependencies within the code under test, you inject them as parameters or properties. In tests, you can provide mock objects or stubs instead of the actual dependencies.
- Constructor injection: Pass dependencies as arguments to the constructor of the class under test.
- Method injection: Pass dependencies as arguments to specific methods of the class under test.
- Property injection: Set dependencies as properties of the class under test.
Dependency injection helps in creating more testable and modular code, as it decouples dependencies and makes it easier to substitute them with mocks or stubs.
Code Coverage and Test Reporting
Understanding Code Coverage
Measuring code coverage can help you assess how much of your code is being exercised by the tests. Code coverage is the measurement of how many lines, branches, or statements in your code are executed during the test suite
Here’s how you can enable code coverage in Jest and Mocha:
1. Jest:
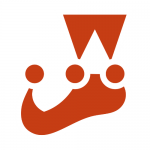
Install Jest and a code coverage tool like istanbul or babel-plugin-istanbul:
npm install jest istanbul –save-dev
Update your package.json to include the following script:
"scripts": {
"test": "jest --coverage"
}
Run your tests with the npm test command, and Jest will automatically generate a code coverage report after the tests complete.
2. Mocha:
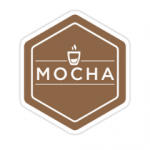
Install nyc, a popular code coverage tool, along with Mocha:
npm install mocha nyc --save-dev
Update your package.json to include the following script:
"scripts": {
"test": "nyc --reporter=html mocha"
}
Run your tests with the npm test command, and Mocha, along with nyc, will generate a code coverage report in HTML format after the tests complete.
Both Jest and Mocha support additional configuration options for code coverage, allowing you to customize the thresholds, specify inclusion/exclusion patterns, and configure report formats. You can refer to their respective documentation for more details on advanced configuration options.
- Generating Code Coverage Reports: Using tools like Istanbul or NYC, you will learn how to generate code coverage reports. To generate code coverage reports in Node.js, you can use tools like nyc, or Jest, which provide built-in support for generating coverage reports. Here’s how you can generate code coverage reports using these tools:
- nyc: nyc is a command-line interface for Istanbul that simplifies code coverage configuration.
install nyc as a development dependency:
npm install nyc --save-dev
Modify your test command in the package.json file to include the nyc command:
"scripts": {
"test": "nyc <test-command>"
}
Replace <test-command> with the command you use to run your tests (e.g., “mocha”).
Run your tests with the modified command:
npm test
nyc will generate a coverage report after running the tests. By default, it will create an HTML report in the coverage directory.
Jest: If you’re using Jest as your testing framework, it has built-in support for generating code coverage reports.
Modify your test command in the package.json file to include the –coverage flag:
"scripts": {
"test": "jest --coverage"
}
Run your tests with the modified command:
npm test
Jest will generate a coverage report after running the tests. It will output the report in the console and also create detailed HTML reports in the coverage directory.
Remember to configure the output format and coverage thresholds according to your requirements. You can refer to the documentation of the specific tool you’re using (Istanbul, nyc, or Jest) for more advanced configuration options, such as generating reports in different formats or excluding specific files from coverage analysis.
Continuous Integration and Test Automation
Integrating Unit Tests into CI/CD Pipelines
Integrating unit tests into your CI/CD (Continuous Integration/Continuous Deployment) pipeline is crucial for ensuring the quality and stability of your Node.js applications.
Here’s a general guide to integrating unit tests into a Node.js CI/CD pipeline:
- Choose a CI/CD Platform: Select a CI/CD platform that suits your needs. Popular options for Node.js projects include Jenkins, CircleCI, Travis CI, GitLab CI/CD, and GitHub Actions.
- Configure the CI/CD Pipeline: Set up a pipeline configuration file (e.g., .yml or .yaml) in your repository’s root directory. The exact configuration format will depend on the chosen CI/CD platform.
- Install Dependencies: Ensure that your CI/CD pipeline installs the necessary dependencies for running tests. Typically, this involves running npm install or yarn install to install the project’s dependencies.
- Run Unit Tests: Configure the pipeline to execute your unit tests. Use the appropriate test runner command for your project, such as npm test, yarn test, or a custom test runner script.
- Generate Code Coverage Reports: If you want to track code coverage, configure the pipeline to generate coverage reports using a code coverage tool like Istanbul or nyc. Specify the appropriate command to generate the coverage reports (e.g., npm test — –coverage or nyc npm test).
- Fail the Build on Test Failures: Ensure that the pipeline fails if any unit tests fail. Configure the pipeline to return a non-zero exit code if tests fail, indicating a failed build.
- Artifact Storage: Optionally, configure the pipeline to store test artifacts such as test reports, code coverage reports, and logs. These artifacts can be useful for later analysis and troubleshooting.
- Trigger the CI/CD Pipeline: Set up triggers for the CI/CD pipeline, such as automatically triggering on each commit or pull request, or based on a schedule.
- Monitor the Pipeline: Regularly monitor the CI/CD pipeline to ensure that it runs successfully and alerts you promptly if any tests fail.
- Integrate with Version Control: Connect your CI/CD pipeline to your version control system, such as Git, to trigger builds and deployments automatically whenever new code is pushed.
It’s important to note that the specific steps and configurations may vary depending on the CI/CD platform you choose. Consult the documentation for your chosen platform to understand its specific configuration options and syntax.

Best Practices for Unit Testing in Node.js
- Writing Testable Code: This section will cover best practices for writing testable code in Node.js. You will learn about the importance of modularization, separation of concerns, and dependency injection to facilitate unit testing.
- Maintaining Independent and Focused Tests: Discover techniques for keeping your tests independent, ensuring that they do not rely on the internal implementation details of the tested units. We will discuss the importance of clear test boundaries and avoiding unnecessary dependencies.
Wrapping Up
In conclusion, unit testing is a vital practice for ensuring the reliability and maintainability of Node.js applications. By following best practices and using the appropriate tools and frameworks, developers can write effective and robust unit tests that help catch bugs early, improve code quality, and support the overall development process. Incorporating unit testing into your development workflow will contribute to building more stable and trustworthy applications.
At ThinkPalm Technologies, we are passionate about software quality and have extensive experience in utilizing best testing practices to create reliable and maintainable applications. Our dedicated software experts leverage unit testing processes to ensure flawless software development services in the UK and worldwide.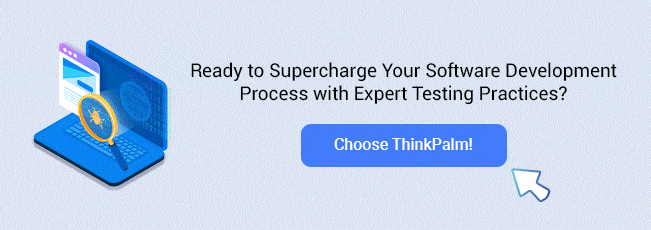
Author Bio
Mukund C Gokulan is an experienced Node.js developer with a deep passion for crafting highly efficient and scalable solutions. With a proven track record of creating robust web applications and APIs using modern JavaScript frameworks and cutting-edge tools, he consistently delivers high-quality code. Mukund's unwavering dedication to optimizing performance compels him to constantly push boundaries and explore innovative approaches, ensuring top-notch user experiences.